Jupyter Notebooks
Contents
Jupyter Notebooks¶
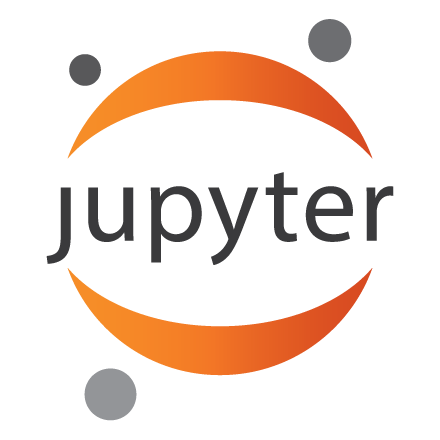
This is a quick introduction to Jupyter notebooks.
Cells¶
Cells, can be markdown (text), like this one or code cells (we’ll get to those).
Markdown cells¶
Markdown cell are useful for communicating information about our notebooks.
They perform basic text formatting including italics, bold, headings, links and images.
Double-click on any of the cells in this section to see what the plain-text looks like. Run the cell to then see what the formatted Markdown text looks like.
This is a heading¶
This is a smaller heading¶
This is a really small heading¶
We can italicize my text either like this or like this.
We can embolden my text either like this or like this.
Here is an unordered list of items:
This is an item
This is an item
This is an item
Here is an ordered list of items:
This is my first item
This is my second item
This is my third item
We can have a list of lists by using identation:
This is an item
This is an item
This is an item
This is an item
This is an item
We can also combine ordered and unordered lists:
This is my first item
This is my second item
This is an item
This is an item
This is my third item
We can make a link to this useful markdown cheatsheet as such.
If we don’t use the markdown syntax for links, it will just show the link itself as the link text: https://www.markdownguide.org/cheat-sheet/
LaTeX-formatted text¶
Code Cells¶
Code cells are cells that contain code, that can be executed.
Comments can also be written in code cells, indicated by ‘#’.
# In a code cell, comments can be typed
a = 1
b = 2
# Cells can also have output, that gets printed out below the cell.
print(a + b)
3
# Define a variable in code
my_string = 'hello world'
# Print out a variable
print(my_string)
hello world
# Operations that return objects get printed out as output
my_string.upper()
'HELLO WORLD'
# Define a list variable
my_list = ['a','b','c']
# Print out our list variable
print(my_list)
['a', 'b', 'c']
Accessing Documentation¶
# Import numpy for examples
import numpy as np
# Check the docs for a numpy array
np.array?
# Check the full source code for numpy append function
np.append??
# Get information about a variable you've created
my_string?
Autocomplete¶
# Move your cursor just after the period, press tab, and a drop menu will appear showing all possible completions
np.
File "<ipython-input-12-06f09a953826>", line 2
np.
^
SyntaxError: invalid syntax
# Autocomplete does not have to be at a period. Move to the end of 'ra' and hit tab to see completion options.
ra
# If there is only one option, tab-complete will auto-complete what you are typing
ran
Kernel & Namespace¶
You do not need to run cells in order! This is useful for flexibly testing and developing code.
The numbers in the square brackets to the left of a cell show which cells have been run, and in what order.
However, it can also be easy to lose track of what has already been declared / imported, leading to unexpected behaviour from running cells.
The kernel is what connects the notebook to your computer behind-the-scenes to execute the code.
It can be useful to clear and re-launch the kernel. You can do this from the ‘kernel’ drop down menu, at the top, optionally also clearing all ouputs.
Magic Commands¶
Magic commands are designed to succinctly solve various common problems in standard data analysis. Magic commands come in two flavors: line magics, which are denoted by a single % prefix and operate on a single line of input, and cell magics, which are denoted by a double %% prefix and operate on multiple lines of input.
# Access quick reference sheet for interactive Python (this opens a reference guide)
%quickref
# Check a list of available magic commands
%lsmagic
# Check the current working directory
%pwd
# Check all currently defined variables
%who
# Chcek all variables, with more information about them
%whos
# Check code history
%hist
Line Magics¶
Line magics use a single ‘%’, and apply to a single line.
# For example, we can time how long it takes to create a large list
%timeit list(range(100000))
Cell Magics¶
Cell magics use a double ‘%%’, and apply to the whole cell.
%%timeit
# For example, we could time a whole cell
a = list(range(100000))
b = [n + 1 for n in a]
Running terminal commands¶
Another nice thing about notebooks is being able to run terminals commands
# You can run a terminal command by adding '!' to the start of the line
!pwd
# Note that in this case, '!pwd' is equivalent to line magic '%pwd'.
# The '!' syntax is more general though, allowing you to run anything you want through command-line
%%bash
# Equivalently, (for bash) use the %%bash cell magic to run a cell as bash (command-line)
pwd
# List files in directory
!ls
# Change current directory
!cd .